Try our AI Code Generators in other Programming Languages
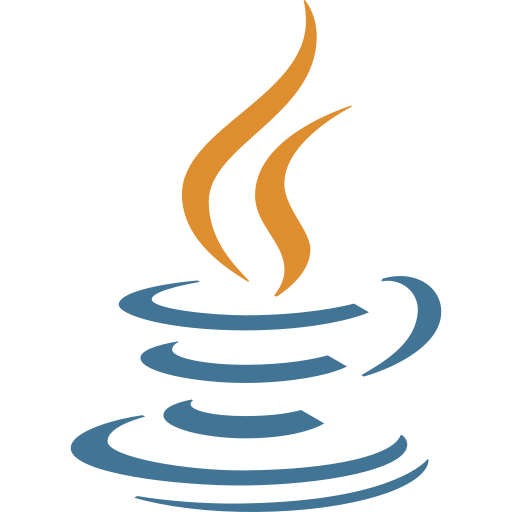
Java
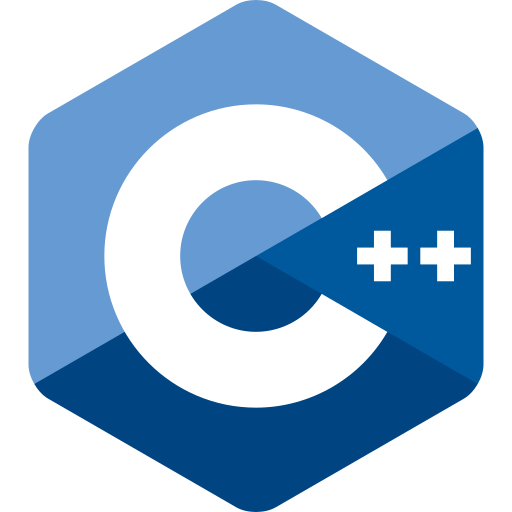
C++
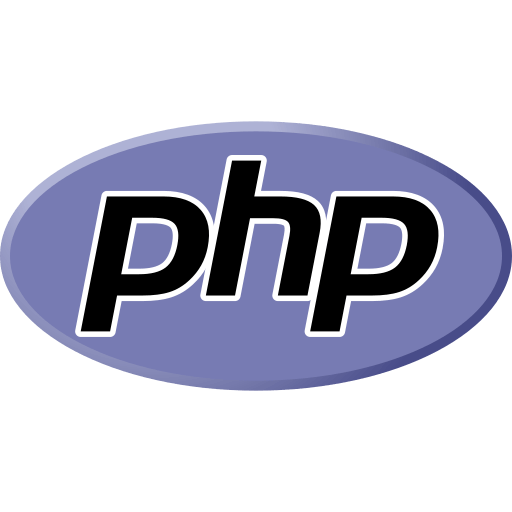
PHP
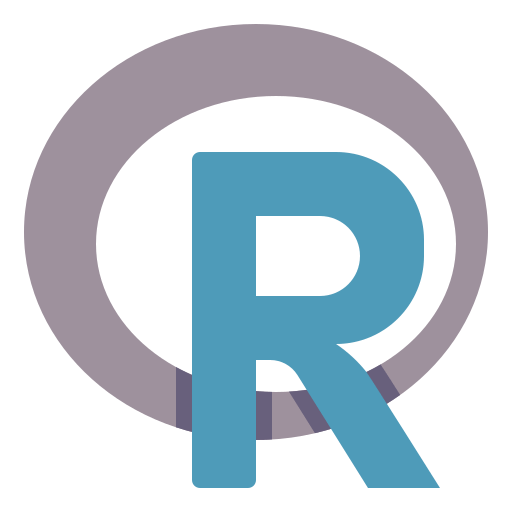
R
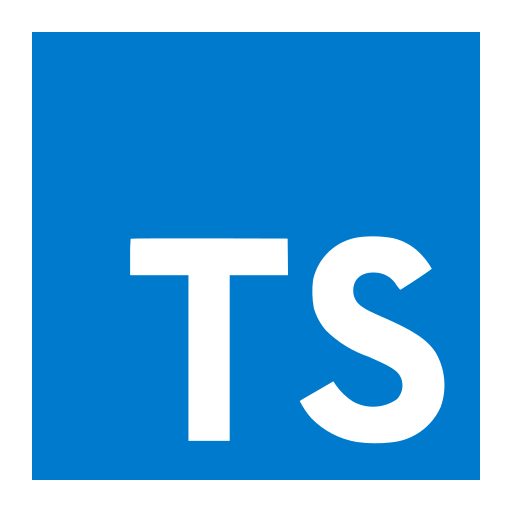
TypeScript
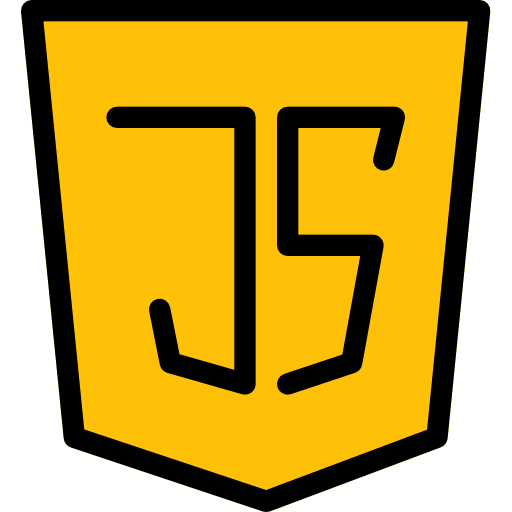
JavaScript
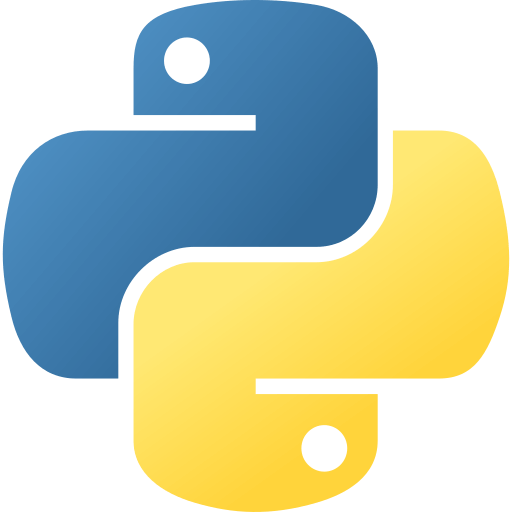
Python
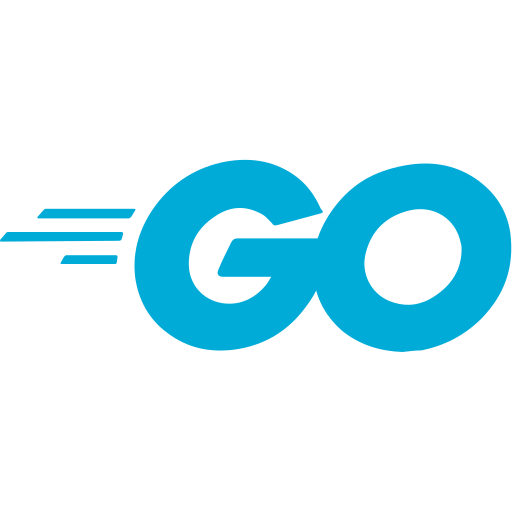
Golang
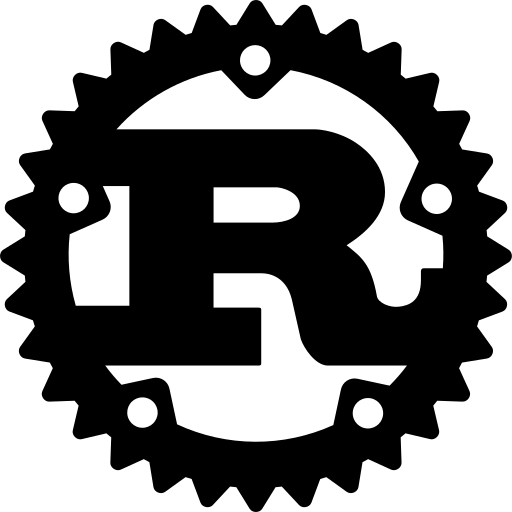
Rust
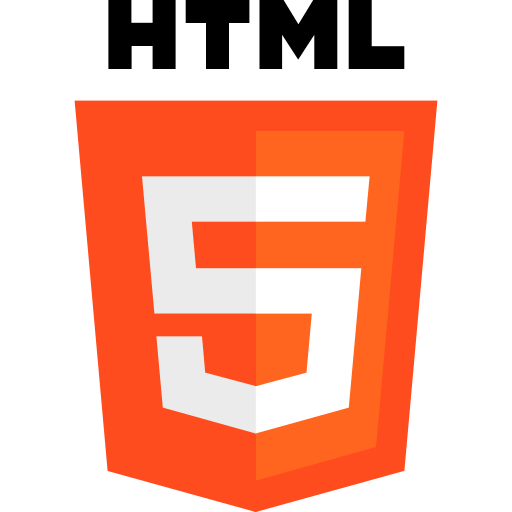
HTML
Still Have Doubts?
We have expert tutors available 24/7 to assist you if you still have any doubts or need more explanations about the code you have. Connect with them online here: